.NET Core code coverage on Linux with MiniCover
Code below can be found in GitHub SampleDotNetCore2RestStub repository. In Code coverage of .NET Core unit tests with OpenCover post, I have shown how to do code coverage with OpenCover. Commands shown in that post can be made part of your CI or CD build. There is a but though, this works only for windows. If you are having build machines on Linux you need another alternative. In this post, I’m going to show this alternative.
MiniCover
MiniCover is a lightweight code coverage tool for .NET Core on Linux. It is in an early stage yet and there is no big community, but I really hope this is going to change soon as it looks a very promising tool.Include in project
In order to use MiniCover it has to be installed as .NET CLI Tool. This is done with following code:<ItemGroup>
<DotNetCliToolReference Include="MiniCover" Version="2.0.0-ci-*" />
</ItemGroup>
In order to keep your original projects intact, the best approach is to create tools project and add it to its tools.csproj file, which will look:
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netcoreapp2.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<DotNetCliToolReference Include="MiniCover" Version="2.0.0-ci-*" />
</ItemGroup>
</Project>
Commands
At this stage following command line options are available:- instrument - Instrument assemblies
- uninstrument - Uninstrument assemblies
- reset - Reset hits count
- report - Outputs coverage report
- htmlreport -Write HTML report to a folder
- xmlreport - Write an NCover-formatted XML report to folder
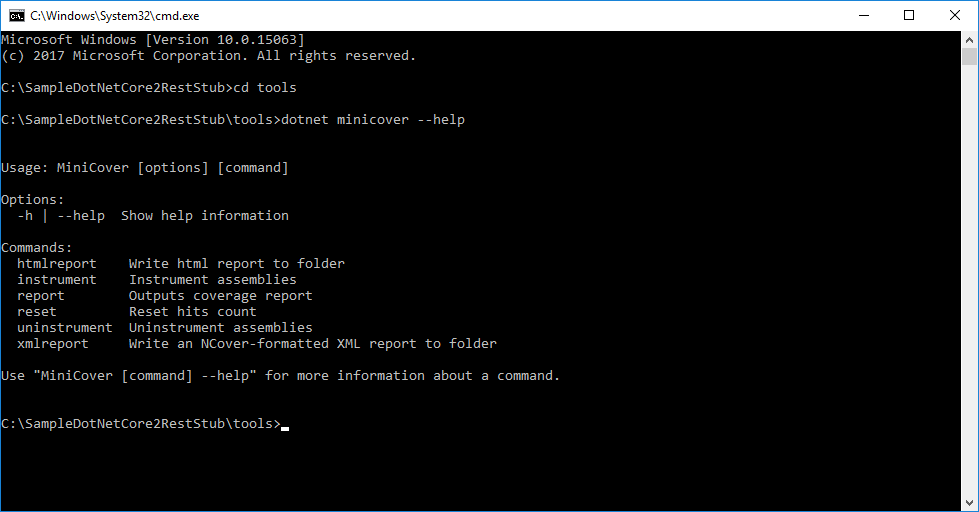
Run coverage
In case of a project structure where you have your code in src folder and your tests in test folder following bash script can be used directly. It accepts as parameter threshold coverage percentage, if not provided it uses 80% by default. Script restores NuGet packages and builds the projects. It navigates to tools folder and restores NuGet packages again. This is very important as it is the only way to get MiniCover NuGet package. Inside tools folder, it instruments assemblies and resets previous statistics. Script navigates to root folder and runs all tests inside every project in test folder. Afterward, script navigates again to tools folder and uninstruments all assemblies so far. No matter this operation is safe I would recommend to run one more build or publish before assemblies go into production. In the end, the script generates reports.if [ ! -z $1 ]; then
if [ $1 -lt 0 ] || [ $1 -gt 100 ]; then
echo "Threshold should be between 0 and 100"
threshold=80
fi
threshold=$1
else
threshold=80
fi
dotnet restore
dotnet build
cd tools
dotnet restore
# Instrument assemblies inside 'test' folder to detect hits for source files inside 'src' folder
dotnet minicover instrument --workdir ../ --assemblies test/**/bin/**/*.dll --sources src/**/*.cs
# Reset hits count in case minicover was run for this project
dotnet minicover reset
cd ..
for project in test/**/*.csproj; do dotnet test --no-build $project; done
cd tools
# Uninstrument assemblies, it's important if you're going to publish or deploy build outputs
dotnet minicover uninstrument --workdir ../
# Create HTML reports inside folder coverage-html
# This command returns failure if the coverage is lower than the threshold
dotnet minicover htmlreport --workdir ../ --threshold $threshold
# Print console report
# This command returns failure if the coverage is lower than the threshold
dotnet minicover report --workdir ../ --threshold $threshold
# Create NCover report
dotnet minicover xmlreport --workdir ../ --threshold $threshold
cd ..
Reports
There are 3 types of reports: Console, HTML and NCover XML.Console report
Console report is dumping results to the console and returns 1 if given threshold is not met, which basically fails the CI/CD build. In the example below, codeCoverage.sh was called with argument 40, which means threshold is 40%.HTML report
HTML report is also failing the build and gives similar summary information as console report, but also give detailed information for each class coverage. An example report can be found in MiniCover HTML report. I have to praise myself, as the summary file that is shown below was something I contributed, because I like the project very much.NCover report
NCover report creates XML file in its format. The beauty of it is that you can additionally use ReportGenerator on Windows machine and convert XML to nice HTML report. Assuming ReportGenerator is extracted on your C:\ then the command is shown below. The report can be found in MiniCover ReportGenerator report.C:\ReportGenerator\ReportGenerator.exe -reports:coverage.xml -targetdir:coverage